Ultimate Design Patterns - Structural Flashcards
What problem does the Composite Pattern solve?

Allows us to work with objects or groups as if they were the same
without having to use Object base class and explicitly cast objects to types
When do we use the Composite Pattern?

Want to represent a hierarchy and treat the objects in hieararchy in the same way (parts and containers)
Ex. Files and Folders

How can we implement the Component Pattern?

Allows us to represents object hierarchies where individual objects and compositions of objects are treated the same way

What’s an excercise using the Composite Pattern?

Represents object hierarchies where individual objects and compositions of objects are treated the same way.

AP What is the Adapter Pattern?

Allows converting the interface of a class into another interface that clients expect.
Just like an electricity adapter converts plug to format!
Ex. Apply various filters to a photo
What real world problem does the Adapter Pattern Solve?
We have a class that we want to use but the interface doesn’t match form we expect
Adapter pattern converts interface of the class to the proper form
We have a third party filter library that doesn’t implement Filter Interface
Our viewImage class only accepts filters that Implement Filter Interface
Adapter Pattern allows us to change third party filter Interface to match required form for viewImage (Filter Interface)

How do we implement the Adapter Pattern using composition?

Favor composition over inheritance!

How do we implement the Adapter Pattern using inheritance?

Not the favorable approach!
Why?
It is less flexible than composition

What is an excercise using the Adapter Pattern?

What problem does the Decorator Pattern solve?

Allows us to add additional behavior to an object dynamically
Why?
For every feature we are adding, have to create various classes to combine features.
With five features, would have to create many classes to combine features!
This isn’t extensible approach.
Ex. We can create a CloudStream object and decorate it with additional behavior

What is the Decorator Pattern?

Allows us to add additional behavior to an existing object

What is an old saying?

Favor composition over inheritence!
Why?
It doesn’t mean inheritance is bad, it’s a great solution to many problems but not this problem

What is similar and different between the Decorator Pattern and the Adapter Pattern?

Both pattern implementation uses composition relationship
Adapter Pattern - We change the interface to a different form
Decorater Pattern - We add additional behavior to an object

How do we implement the Decorator Pattern?

Allows us to add additional operations to an object
How?
Decorator - a wrapper class with additional operations composed of an object

What is an excercise using the Decorator Pattern?

What do we use the Facade Pattern to do?

Provide a simple interface to a complex system
What is the problem with this implementation?

- Sending a notification is difficult - Everytime we want to send a push notification to a user we have to follow a number of steps! We have to create new notification server, connect, create message, send it!
- Coupling - Main class is coupled to four classes. If another class wants to send a message have to also couple to these four classes. If a future change, have to recompile them all!
Solution?
Facade Pattern

How do we implement the Facade Pattern?


What is an excercise using Facade Pattern?
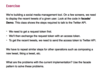

When do we use the Flyweight Pattern?

Reduce amount of memory consumed by objects
Ex. GoogleMaps - rending maps has cafes, restaurants, schools.
A mobile phone trying to render large point objects will run out of memory and crash!
What is the problem with this implementation?

On some mobile devices application freezes or crashes
Why?
The point class consumes a fair amount of memory
We could have thousands of points
With this structure, Cafe Icon (20KB) will be stored 100 times (20MB)
We can store it only once in memory and use it across multiple point objects!
Ex. Each pt is 20MB * 100 pts = 20 KB memory!
Many users have Apps in background not closed, using memory!

What is the flyweight pattern?
Allows objects to cache data that is shared across multiple objects to reduce memory!
Why?
Allows to reduce memory use for objects
How?
Separate the data we need to share
store it in Flyweight class (object we can share)
implement a factory for caching these objects

What is an excercise using the Flyweight Pattern?


What problem does the Bridge Pattern solve?

Building a simple, yet flexible hierarchy
for a hierarchy that can grow in 2 dimensions










