TDD Flashcards
TDD steps
It has four steps:
- Write a failing test
- Make the test pass
- Refactor
- Repeat
Red: Write a failing test, before writing any app code.
Green: Write the bare minimum code to make the test pass.
Refactor: Clean up both your app and test code.
Repeat: Do this cycle again until all features are implemented.

Test conventions
- XCTest: Requires all test methods begin with test to be run.
- test: Followed by the name of the method being tested. Here, this is init. There’s then an underscore to separate it from the next part.
Optionally, if special set up is required, this comes next. This test doesn’t include this. If provided, this likewise is followed by an underscore to separate it from the last part.
Lastly, this is followed by the expected outcome or result. Here this is createsCashRegister.
A few things you might look to refactor in refactor state in TDD
Duplicate logic
Comments
Code smells
Three parts of test
Given a certain condition…
When a certain action happens…
Then an expected result occurs.
In which order are test methods executed?
The order in which test classes and test methods are run is not explicitly defined and cannot be relied upon.
Lifecycle of XCTestCase
XCTestCase subclass lifecycles are managed outside the test execution, and any class-level state is persisted between test methods.
When setUp() and tearDown() are executed?
After and before each test
Organisation of test target folder

@testable attribute
Xcode provides a way to expose data types for testing without making them available for general use
This makes symbols that are open, public, and internal available to the test case.
Not compiling counts as a failure?
yes
Use tests to guide … what?
Use tests to guide refactoring code for readability and performance.
Functonal view controller testing is done by?
Functional testing is done by using separate methods for interacting with the UI (callbacks, delegate methods, etc.) from logic methods (updating state).
Using the host app
you have access to the UIApplication object and the whole View hierarchy in the tests.
One alternate way of retrieving and testing a view controller can be done as follows: First, get a reference to the storyboard:

Turn on Randomized order

Code coverage
Coverage doesn’t mean the code works, but lack of coverage means that it’s not tested. For views and view controllers, it’s not expected to get to 100% coverage because TDD does not include UI testing. When you combine unit tests with UI automation tests, then you should expect to be able to cover most if not all of these files.
Debugging tests
Make sure:
You have the right assumptions in the given statements.
Your then statements accurately reflect the desired behavior.
Using test breakpoints

XCTest expectations have two parts:
the expectation and a waiter. An expectation is an object that you can later fulfill. The wait method of XCTestCase tells the test execution to wait until the expectation is fulfilled or a specified amount of time passes.
Testing for true asynchronicity
Create custom class that observe if text/color/font changes when “when” part happens in test and fulfill expectation within this class

What expectation can expect?
NotificationCenter
exp.fulfill()
pro
expectedFulfillmentCount
You can use
expectedFulfillmentCount to set how many times you have to fulfill exp
Expecting something not to happen
exp.isInverted = true
you can invert expectation
What you can add to Notification
?
You can add data through userInfo[:]
Expectation can be set. How?


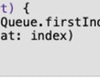




