Chap 2 - PART 1 - Language of the Computer Flashcards
Image showing a Simplified Overview of compiling your program

Image:
Assembly language

List the characteristics of the assembly language:
RISC
RISC: Reduced Instruction Set Computer
- Provides a small set of instructions, each designed to attain a small task
- The programmer may need to use more instructions to complete some given task
- Less straining on the hardware
- eg. MIPS, ARM, RISC-V
List the characteristics of the assembly language:
CISC
CISC: Complex Instruction Set Computer
- Provides instructions skilled in executing multi-step operations
- Same task can be achieved using less instructions than RISC
- Difficult to implement on the hardware
- eg. x86
Image:
Table showing the differences between RISC vs CISC

Describe the arithmetic operations:
State: Design Principle 1

Arithmetic Example:
- C Code:
f = (g+h) - (i+j);
- Compile the code above to MIPS Code

Describe: Registers
- This refers to the small bit of memory in the cetral processing unit (CPU)
- It is used in assembly language to perform different instructions
- Limited number of special locations built directly into the hardware
- Operations can only be performed on these; registers are the tiniest, fastest, and most expensive type of memory
Benefit:
- Since registers are directly in hardware, they are very fast (faster than 0.25ns)
- Unlike HLL like C or Java, assembly cannot use variables
- why not? find out? maybe to keep hardware simple?
Image:
Registers inside the processor
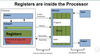
- State the drawback of registers:
- State the solution for this drawback:
- Why are there 32 registers only in MIPS
- What is a group of 32 bits called?
- Since registers are in hardware, there is a predetermined number of them, hence, limited.
- The solution is that MIPS code must be very carefully put together to efficiently use registers. In other words, the power is in the hand of the programmer.
- 32 bits because smaller is faster, however, too small is bad
- group of 32 bits is called a word. EACH MIPS register is only 32 bits wide.
Describe:
the Constant Zero
MIPS register 0 ($zero) is known as the constant zero
- it cannot be overwritten
Useful for common operations
- eg. Move between registers
- add $t2, $s1, $zero
Image:
Register usage

About register operands:
- what types of instructions uses register operands?
- Describe the characteristics of MIPS that has a 32x32 bit register file
- arithmetic instructions
- Use for frequently accessed data ; numbered 0 to 31
List the Assembler names for register operands
$t0, $t1, … $t9, for temporary values
$s0, $s1, …, $s7, for saved variables.
State:
the design principle 2
Smaller is faster:
- c.f. main memory: millions of location
Register Operand Example:
- C code:
- f = ( g + h ) - ( i + j );
- f, …., j in $s0, …, $s4
- Compile the code above in MIPS

List the main memory that is used for composite data
- arrays
- structures
- dynamic data
- How to apply arithmetic operations? What are the steps?
- Load values from memory into registers
- Store result from register to memory
Memory is byte addressed, each address identifies an?
each address identifies an 8 bit style
Words are aligned in memory, therefore, address must be a multiple of?
4
MIPS is Big ____
why?
Endian
because most significant byte at lowest address of a word
Memory Operand Example 1
C code:
- g = h + A[8];
- g in $s1, h in $s2, base address of A in $s3
Compile the code above in MIPS code:
- Note that: index 8 requires offset of 32
- There are 4 bytes per word

Memory Operand Example 2
Code:
- A[12] = h + A[8];
- h in $s2, base address of A in $s3
Compile the code above in MIPS code:
- Note that: Index 8 requires offset of 32

Describe:
the differences between Register vs Memory
- Registers are faster to access THAN memory
- operating on memory datra requires loads and stores
- thus, more instructions to be executed!!!!
- Compiler must use registers for variables as much as possible
- only spill to memory for less frequently used vars
- Remember that register optimization is important!!!














